.NETのReflection(リフレクション)を使ったTipsのコードスニペット集です。
本記事に記載のコードでは、以下のusingディレクティブの記述が必要になります。
1 2 3 4 5 6 7 8 9 10 11 12 |
// using ディレクティブ using System; using System.Collections; using System.Collections.Generic; using System.Linq; using System.Data; using System.Reflection; using System.Windows.Forms; using System.Drawing; using System.Text; using System.IO; using System.Xml; |
本文中では、usingの記述を省略しています。
本記事では、以下のユーザー定義クラスを使用します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
// スーパークラス(親クラス) private class SuperClass { public string BaseProperty { get; set; } public void BaseMethod() { Console.WriteLine("Hello World!"); } } // サブクラス(子クラス) private class SubClass : SuperClass { public string DerivedProperty { get; set; } public void DerivedMethod() { Console.WriteLine("Hello World!"); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 |
private class MyClass { public MyClass() { } public MyClass(string name) { Name = name; } public MyClass(string name, int value) { Value = value; } public string Name { get; set; } public int Value { get; set; } public object PropertyForGet { get; } private object _propertyForSet; public object PropertyForSet { set { _propertyForSet = value; } } public long? NullableProperty { get; set; } public void WriteName() { Console.WriteLine($"My name is {Name}"); } public void WriteNameAndValue(string name, int value) { Console.WriteLine($"Name: {name}, Value: {value}"); } public string GetTextValue() { return "Return text value."; } } private class YourClass { public YourClass() { } private int nonPublicField; public string PublicField; internal decimal NonPublicProperty { get; set; } public DateTime PublicProperty { get; set; } protected void NonPublicMethod() { Console.WriteLine("NonPublicMethod"); } public void PublicMethod() { Console.WriteLine("PublicMethod"); } public static char StaticField; public static short StaticProperty => short.MaxValue; public static void StaticMethod() { Console.WriteLine("StaticMethod"); } public static void StaticMethodWithParameters(string param1, int param2) { Console.WriteLine($"Param1: {param1}, Param2: {param2}"); } } [Serializable] private class OurClass { public OurClass() { } [IsCustom] public int OurProperty { get; set; } [Obsolete("This method is no longer used.", true)] public void OurMethod(params string[] values) { foreach (string value in values) { Console.WriteLine(value); } } } [AttributeUsage(AttributeTargets.Property)] private sealed class IsCustomAttribute : Attribute { } |
.NETを用いて作成されているプログラムやライブラリーなどのオブジェクトが持つ情報にアクセスし、データを取得、設定したり、作成したり、実行したりできる「メタデータ」を操作するための仕組みです。
リフレクションを使うと、アセンブリや、アセンブリに定義されているクラス、インターフェース、構造体、列挙体などに関する情報を取得したり、プログラムの実行時に型のインスタンスを作成したり、作成した型インスタンスを呼び出したり、アクセスしてデータを設定したりすることができます。
リフレクションはオブジェクトの型情報をもとに操作します。
目次
- 1 型オブジェクト(Type型のインスタンス)を取得する
- 2 型の名前を取得する
- 3 型のフルネームを取得する
- 4 型のアセンブリ修飾名を取得する
- 5 型の名前空間を取得する
- 6 型のTypeCodeを取得する
- 7 オブジェクトが指定した型と同一かどうかを判定する
- 8 オブジェクトが指定した型に代入できるかどうかを判定する
- 9 配列かどうかを判定する
- 10 ジェネリック型かどうかを判定する
- 11 ジェネリック型の型パラメーター(型引数)の型を取得する
- 12 ジェネリック型を構築する元になるジェネリック型の型を取得する
- 13 値型かどうかを判定する
- 14 クラスかどうかを判定する
- 15 ジェネリッククラスかどうかを判定する
- 16 List<T>型かどうかを判定する
- 17 インターフェースかどうかを判定する
- 18 プリミティブ型かどうかを判定する
- 19 非ジェネリックコレクションかどうかを判定する
- 20 列挙型(enum)かどうかを判定する
- 21 列挙型(enum)の値に対応する列挙子(フィールド名)を取得する
- 22 列挙型(enum)の列挙子(フィールド名)の一覧を取得する
- 23 列挙型(enum)に定義されている値かどうかを判定する
- 24 構造体かどうかを判定する
- 25 型のアセンブリを取得する
- 26 アセンブリのフルネームを取得する
- 27 アセンブリの場所を取得する
- 28 アセンブリ名の詳細を取得する
- 29 アセンブリのバージョンを取得する
- 30 アセンブリの簡易名を取得する
- 31 アセンブリに定義されている型を取得する
- 32 アセンブリに含まれるpublicな型を取得する
- 33 実行中のコードを含むアセンブリを取得する
- 34 実行中のエントリポイントを含むアセンブリを取得する
- 35 アセンブリ名からアセンブリをロードする
- 36 ファイル名(パス)からアセンブリーをロードする
- 37 アセンブリ内のクラスのインスタンスを生成する
- 38 アセンブリのモジュール(dll/exe)を取得する
- 39 型のモジュール(dll/exe)を取得する
- 40 引数なしのコンストラクターを取得する
- 41 引数なしのコンストラクターからインスタンスを生成する
- 42 引数ありのコンストラクターを取得する
- 43 引数ありのコンストラクターからインスタンスを生成する
- 44 親クラス(ベースクラス)の型を取得する
- 45 特定のクラスのサブクラス(派生クラス)かどうかを判定する
- 46 実装しているインターフェースの一覧を取得する
- 47 パブリックフィールドを取得する
- 48 パブリックプロパティを取得する
- 49 パブリックメソッドを取得する
- 50 インスタンスフィールドを取得する
- 51 インスタンスプロパティを取得する
- 52 インスタンスメソッドを取得する
- 53 静的フィールドを取得する
- 54 静的プロパティを取得する
- 55 静的メソッドを取得する
- 56 非パブリックフィールドを取得する
- 57 非パブリックプロパティを取得する
- 58 非パブリックメソッドを取得する
- 59 現在実行されているメソッドを取得する
- 60 メソッド名を取得する
- 61 メソッドが定義されているクラスを取得する
- 62 クラスで定義されたメソッドのみ取得する
- 63 特定のフィールドを取得する
- 64 特定のプロパティを取得する
- 65 特定のメソッドを取得する
- 66 インスタンス・メソッドを呼び出す(引数なし)
- 67 インスタンス・メソッドを呼び出す(引数あり)
- 68 静的メソッドを呼び出す(引数なし)
- 69 静的メソッドを呼び出す(引数あり)
- 70 メソッドのパラメーター(引数)を取得する
- 71 メソッドの戻り値を取得する
- 72 メソッドの戻り値の型を取得する
- 73 プロパティの型を取得する
- 74 プロパティの値を取得する
- 75 プロパティに値を設定する
- 76 読み取り可能なプロパティかどうかを判定する
- 77 書き込み可能なプロパティかどうかを判定する
- 78 プロパティがNull許容型(Nullable<T>型)かどうかを判定する
- 79 指定した属性が関連付けられているかどうかを判定する
- 80 型に関連付けられている属性を取得する
- 81 型に関連付けられているカスタム属性を含むコレクションを取得する
- 82 型に関連付けられている特定の型の属性を取得する
- 83 プロパティに関連付けられている属性を取得する
- 84 プロパティに関連付けられているカスタム属性を含むコレクションを取得する
- 85 プロパティに関連付けられている特定の型の属性を取得する
- 86 メソッドに関連付けられている属性を取得する
- 87 メソッドに関連付けられているカスタム属性を含むコレクションを取得する
- 88 メソッドに関連付けられている特定の型の属性を取得する
- 89 型のメンバーを取得する
- 90 名前を指定して型のメンバーを取得する
- 91 メンバーの種類を取得する
- 92 すべてのフィールドを取得する
- 93 すべてのプロパティを取得する
- 94 すべてのメソッドを取得する
- 95 すべてのイベントを取得する
型オブジェクト(Type型のインスタンス)を取得する
リフレクション(Reflection)を使用するには、まず型のオブジェクトを取得します。
型オブジェクト(System.Type型のインスタンス)を取得(生成)する方法はいくつかあります。
1 2 3 4 5 6 7 8 9 |
// typeof演算子で型を取得 Type typeOfStringBuilder = typeof(StringBuilder); // TypeクラスのGetTypeメソッドで型を取得 Type typeOfStringBuilder = Type.GetType("System.Text.StringBuilder, mscorlib, Version = 4.0.0.0, Culture = neutral, PublicKeyToken = b77a5c561934e089"); // インスタンスのGetTypeメソッドで型を取得 StringBuilder stringBuilder = new StringBuilder(); Type typeOfStringBuilder = stringBuilder.GetType(); |
オブジェクトの型オブジェクトを取得する方法の詳細については、以下の記事を参考にしてください。
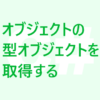
型の名前を取得する
型の名前を取得するには、TypeクラスのNameプロパティを使用します。
1 2 3 |
Type type = typeof(decimal); Console.WriteLine(type.Name); // Decimal |
型のフルネームを取得する
型のフルネームを取得するには、TypeクラスのFullNameプロパティを使用します。
1 2 3 |
Type type = typeof(DateTime); Console.WriteLine(type.FullName); // System.DateTime |
型のアセンブリ修飾名を取得する
型のアセンブリ修飾名を取得するには、TypeクラスのAssemblyQualifiedNameプロパティを使用します。
1 2 3 |
Type type = typeof(StringBuilder); Console.WriteLine(type.AssemblyQualifiedName); // System.Text.StringBuilder, mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089 |
型の名前空間を取得する
型の名前空間を取得するには、TypeクラスのNamespaceプロパティを使用します。
1 2 3 |
Type type = typeof(Form); Console.WriteLine(type.Namespace); // System.Windows.Forms |
型のTypeCodeを取得する
型のTypeCodeを取得するには、TypeクラスのTypeCode静的メソッドを使用します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
TypeCode code = Type.GetTypeCode(DateTime.Today.GetType()); Console.WriteLine(code); Func<object, string> getTypeName = obj => { Type type = obj.GetType(); TypeCode typeCode = Type.GetTypeCode(type); switch (typeCode) { case TypeCode.Boolean: return "bool"; case TypeCode.Byte: return "byte"; case TypeCode.Char: return "char"; case TypeCode.DateTime: return "DateTime"; case TypeCode.DBNull: return "DBNull"; case TypeCode.Decimal: return "decimal"; case TypeCode.Double: return "double"; case TypeCode.Empty: return "Empty"; case TypeCode.Int16: return "short"; case TypeCode.Int32: return "int"; case TypeCode.Int64: return "long"; case TypeCode.Object: return "object"; case TypeCode.SByte: return "sbyte"; case TypeCode.Single: return "float"; case TypeCode.String: return "string"; case TypeCode.UInt16: return "ushort"; case TypeCode.UInt32: return "uint"; case TypeCode.UInt64: return "ulong"; default: return "unknow"; } }; Console.WriteLine(getTypeName("abcde")); // string Console.WriteLine(getTypeName(123.45)); // double Console.WriteLine(getTypeName(DayOfWeek.Monday)); // int Console.WriteLine(getTypeName(new Form())); // object |
オブジェクトが指定した型と同一かどうかを判定する
オブジェクトが指定した型と同一かどうかを判定するには、==演算子を使用します。
1 2 3 4 5 6 7 |
SuperClass superClass = new SuperClass(); SubClass subClass = new SubClass(); Console.WriteLine(superClass.GetType() == typeof(SuperClass)); // True Console.WriteLine(subClass.GetType() == typeof(SuperClass)); // False |
オブジェクトが指定した型に代入できるかどうかを判定する
オブジェクトが指定した型に代入できるかどうかを判定するには、is演算子を使用します。
1 2 3 4 5 6 7 |
SuperClass superClass = new SuperClass(); SubClass subClass = new SubClass(); Console.WriteLine(subClass is SuperClass); // True Console.WriteLine(subClass is SubClass); // True |
配列かどうかを判定する
配列かどうかを判定するには、TypeクラスのIsArrayプロパティを使用します。
1 2 3 4 |
int[] array = new int[256]; Type type = array.GetType(); Console.WriteLine(type.IsArray); // True |
ジェネリック型かどうかを判定する
ジェネリック型かどうかを判定するには、TypeクラスのIsGenericTypeプロパティを使用します。
1 2 3 4 |
IEnumerable<int> enumerable = new List<int>(); Type type = enumerable.GetType(); Console.WriteLine(type.IsGenericType); // True |
ジェネリック型の型パラメーター(型引数)の型を取得する
ジェネリック型の型パラメーター(型引数)の型を取得するには、TypeクラスのGetGenericArgumentsメソッドを使用します。
1 2 |
Type type = typeof(Func<int, int, long>); Type[] types = type.GetGenericArguments(); |
ジェネリック型を構築する元になるジェネリック型の型を取得する
ジェネリック型を構築する元になるジェネリック型の型を取得するには、TypeクラスのGetGenericTypeDefinitionメソッドを使用します。
1 2 3 4 |
Type type = typeof(Action<string, int, int>); Type GetGenericTypeDefinition = type.GetGenericTypeDefinition(); Console.WriteLine(GetGenericTypeDefinition); // System.Action`3[T1,T2,T3] |
値型かどうかを判定する
値型かどうかを判定するには、TypeクラスのIsValueTypeプロパティを使用します。
1 2 3 |
Type type = typeof(long); Console.WriteLine(type.IsValueType); // True |
クラスかどうかを判定する
クラスかどうかを判定するには、TypeクラスのIsClassプロパティを使用します。
1 2 3 |
Type type = typeof(DataSet); Console.WriteLine(type.IsClass); // True |
ジェネリッククラスかどうかを判定する
ジェネリッククラスかどうかを判定するには、TypeクラスのIsGenericTypeプロパティと、TypeクラスのIsClassプロパティを組み合わせて使用します。
1 2 3 |
List<int> list = new List<int>(); Type type = list.GetType(); Console.WriteLine(type.IsClass && type.IsGenericType); |
List<T>型かどうかを判定する
List<T>型かどうかを判定するには、TypeクラスのGetGenericTypeDefinitionメソッドで取得した型が、List<>型であるかどうかを調べます。
1 2 3 4 5 6 |
List<string> list = new List<string>(); Type type = list.GetType(); if (type.GetGenericTypeDefinition() == typeof(List<>)) { Console.WriteLine("List<T>"); } |
インターフェースかどうかを判定する
インターフェースかどうかを判定するには、TypeクラスのIsInterfaceプロパティを使用します。
1 2 |
Type type = typeof(IList<>); Console.WriteLine(type.IsInterface); |
プリミティブ型かどうかを判定する
プリミティブ型かどうかを判定するには、TypeクラスのIsPrimitiveプロパティを使用します。
1 2 3 4 |
Console.WriteLine(typeof(int).IsPrimitive); // True Console.WriteLine(typeof(string).IsPrimitive); // False |
非ジェネリックコレクションかどうかを判定する
非ジェネリックコレクションかどうかを判定するには、TypeクラスのIsAssignableFromメソッドを使用して型が、IEnumerable型に割り当てできるかどうかを検査し、尚且つ文字列(string)型でないかどうかを調べます。
1 2 3 |
Dictionary<string, object> keyValuePairs = new Dictionary<string, object>(); Type type = keyValuePairs.GetType(); Console.WriteLine(typeof(IEnumerable).IsAssignableFrom(type) && type != typeof(string)); |
列挙型(enum)かどうかを判定する
列挙型(enum)かどうかを判定するには、TypeクラスのIsEnumプロパティを使用します。
1 2 |
Type type = typeof(DayOfWeek); Console.WriteLine(type.IsEnum); |
列挙型(enum)の値に対応する列挙子(フィールド名)を取得する
列挙型(enum)の値に対応する列挙子(フィールド名)を取得するには、TypeクラスのGetEnumNameメソッドを使用します。
1 2 3 |
Type type = typeof(DayOfWeek); Console.WriteLine(type.GetEnumName(3)); // Wednesday |
列挙型(enum)の列挙子(フィールド名)の一覧を取得する
列挙型(enum)の列挙子(フィールド名)の一覧を取得するには、TypeクラスのGetEnumNamesメソッドを使用します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
Type type = typeof(DayOfWeek); foreach (string name in type.GetEnumNames()) { Console.WriteLine(name); } /* Sunday Monday Tuesday Wednesday Thursday Friday Saturday */ |
列挙型(enum)に定義されている値かどうかを判定する
列挙型(enum)に定義されている値かどうかを判定するには、TypeクラスのIsEnumDefinedメソッドを使用します。
1 2 3 4 5 |
Type type = typeof(DayOfWeek); Console.WriteLine(type.IsEnumDefined(4)); // True Console.WriteLine(type.IsEnumDefined(7)); // False |
構造体かどうかを判定する
構造体かどうかを判定するには、まずTypeクラスのIsValueTypeメソッドで値型どうかを判定します。
値型の場合は同じくTypeクラスのIsPrimitiveメソッド、IsEnumメソッド、IsGenericTypeメソッドで、プリミティブ型ではないか、列挙型ではないか、ジェネリック型ではないかを判定します。
上記すべての条件に合致する場合は構造体になると判断します。
1 2 3 4 5 6 7 8 |
Type type = typeof(decimal); if (type.IsValueType) { if (!type.IsPrimitive && !type.IsEnum && !type.IsGenericType) { Console.WriteLine("Type is struct."); } } |
型のアセンブリを取得する
型のアセンブリを取得するには、TypeクラスのAssemblyプロパティを使用します。
1 2 |
Type type = typeof(DateTime); Assembly assembly = type.Assembly; |
アセンブリのフルネームを取得する
アセンブリのフルネームを取得するには、AssemblyクラスのFullNameプロパティを使用します。
1 2 3 4 |
Type type = typeof(DateTime); Assembly assembly = type.Assembly; Console.WriteLine(assembly.FullName); // mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089 |
アセンブリの場所を取得する
アセンブリの場所を取得するには、AssemblyクラスのLocationプロパティを使用します。
1 2 3 4 |
Type type = typeof(DateTime); Assembly assembly = type.Assembly; Console.WriteLine(assembly.Location); // C:\Windows\Microsoft.NET\Framework\v4.0.30319\mscorlib.dll |
アセンブリ名の詳細を取得する
アセンブリのフルネームを取得するには、AssemblyクラスのGetNameメソッドを使用します。
1 2 3 |
Type type = typeof(DateTime); Assembly assembly = type.Assembly; AssemblyName assemblyName = assembly.GetName(); |
アセンブリのバージョンを取得する
アセンブリのバージョンを取得するには、AssemblyNameクラスのVersionプロパティを使用します。
1 2 3 4 |
Type type = typeof(DateTime); Assembly assembly = type.Assembly; AssemblyName assemblyName = assembly.GetName(); Version version = assemblyName.Version; |
アセンブリの簡易名を取得する
アセンブリのバージョンを取得するには、AssemblyNameクラスのNameプロパティを使用します。
1 2 3 4 5 |
Type type = typeof(DateTime); Assembly assembly = type.Assembly; AssemblyName assemblyName = assembly.GetName(); Console.WriteLine(assemblyName.Name); // mscorlib |
アセンブリに定義されている型を取得する
アセンブリに定義されている型を列挙するには、AssemblyクラスのGetTypesメソッドを使用します。
1 2 3 |
Type type = typeof(FileInfo); Assembly assembly = type.Assembly; Type[] types = assembly.GetTypes(); |
アセンブリに含まれるpublicな型を取得する
アセンブリに含まれるpublicな型を列挙するには、AssemblyクラスのGetTypesメソッドで取得できる型(Type)のうち、TypeクラスのIsPublicプロパティがTrueの型のみを対象にします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
Type type = typeof(XmlDocument); Assembly assembly = type.Assembly; IEnumerable<Type> publicTypes = assembly.GetTypes().Where(t => t.IsPublic); foreach (Type publicType in publicTypes) { Console.WriteLine(publicType.Name); } /* XmlNamedNodeMap IApplicationResourceStreamResolver IHasXmlNode ... 中略 ... XmlPreloadedResolver XmlReaderSection XsltConfigSection */ |
実行中のコードを含むアセンブリを取得する
実行中のコードを含むアセンブリを取得するには、AssemblyクラスのGetExecutingAssembly静的メソッドを使用します。
1 |
Assembly assembly = Assembly.GetExecutingAssembly(); |
実行中のエントリポイントを含むアセンブリを取得する
実行中のエントリポイントを含むアセンブリ(既定のアプリケーション ドメインで実行できるプロセスであるアセンブリ)を取得するには、AssemblyクラスのGetEntryAssembly静的メソッドを使用します。
1 |
Assembly assembly = Assembly.GetEntryAssembly(); |
アセンブリ名からアセンブリをロードする
アセンブリ名からアセンブリを動的にロードするには、AssemblyクラスのLoad静的メソッドを使用します。
1 2 3 |
string fullName = "mscorlib, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089"; AssemblyName assemblyName = new AssemblyName(fullName); Assembly assembly = Assembly.Load(assemblyName); |
ファイル名(パス)からアセンブリーをロードする
ファイル名(パス)からアセンブリーを動的にロードするには、AssemblyクラスのLoadFrom静的メソッドを使用します。
1 2 |
string path = @"C:\Windows\Microsoft.NET\Framework\v4.0.30319\mscorlib.dll"; Assembly assembly = Assembly.LoadFile(path); |
アセンブリ内のクラスのインスタンスを生成する
アセンブリ内のクラスのインスタンスを生成するには、ActivatorクラスのCreateInstance静的メソッドを使用します。
1 2 3 4 |
string path = @"C:\Windows\Microsoft.NET\Framework\v4.0.30319\System.Windows.Forms.dll"; Assembly assembly = Assembly.LoadFile(path); Type type = assembly.GetType("System.Windows.Forms.TextBox"); object instance = Activator.CreateInstance(type); |
クラスのインスタンスはAssemblyクラスのCreateInstanceメソッドで生成することもできます。
1 2 3 |
Type type = typeof(TextBox); Assembly assembly = type.Assembly; object instance = assembly.CreateInstance(type.FullName); |
アセンブリのモジュール(dll/exe)を取得する
アセンブリのモジュール(dll/exe)を取得するには、AssemblyクラスのModulesプロパティを使用します。
1 2 3 |
Type type = typeof(DataTable); Assembly assembly = type.Assembly; Module[] modules = assembly.GetModules(); |
型のモジュール(dll/exe)を取得する
型のモジュール(dll/exe)を取得するには、TypeクラスのModuleプロパティを使用します。
1 2 3 4 |
Type type = typeof(Color); Module module = type.Module; Console.WriteLine(module.Name); // System.Drawing.dll |
引数なしのコンストラクターを取得する
引数なしのコンストラクターを取得するには、TypeクラスのGetConstructorメソッドを使用します。
1 2 |
Type type = typeof(MyClass); ConstructorInfo constructorInfo = type.GetConstructor(Type.EmptyTypes); |
引数なしのコンストラクターからインスタンスを生成する
引数なしのコンストラクターを呼び出してインスタンスを生成するには、ConstructorInfoクラスのInvokeメソッドを使用します。
1 2 3 4 5 |
Type type = typeof(MyClass); ConstructorInfo constructorInfo = type.GetConstructor(Type.EmptyTypes); object instance = constructorInfo.Invoke(null); Console.WriteLine(instance.ToString()); // ConsoleApp1.Program+MyClass |
引数ありのコンストラクターを取得する
引数ありのコンストラクターを取得するには、TypeクラスのGetConstructorメソッドのパラメーター(引数)に、コンストラクターのパラメーター(引数)の型を指定します。
1 2 |
Type type = typeof(MyClass); ConstructorInfo constructorInfo = type.GetConstructor(new Type[] { typeof(string), typeof(int) }); |
引数ありのコンストラクターからインスタンスを生成する
引数ありのコンストラクターを呼び出してインスタンスを生成するには、ConstructorInfoクラスのInvokeメソッドのパラメーター(引数)に、コンストラクターのパラメーター(引数)に指定するオブジェクトを指定します。
1 2 3 4 5 |
Type type = typeof(MyClass); ConstructorInfo constructorInfo = type.GetConstructor(new Type[] { typeof(string), typeof(int) }); object instance = constructorInfo.Invoke(new object[] { "名前", 123 }); Console.WriteLine(instance.ToString()); // ConsoleApp1.Program+MyClass |
親クラス(ベースクラス)の型を取得する
親クラス(ベースクラス)を取得するには、TypeクラスのBaseTypeプロパティを使用します。
1 2 3 4 5 |
SubClass subClass = new SubClass(); Type type = subClass.GetType(); Type baseType = type.BaseType; Console.WriteLine(baseType.FullName); // ConsoleApp1.Program+SuperClass |
特定のクラスのサブクラス(派生クラス)かどうかを判定する
特定のクラスから派生されたかどうかを判定するには、TypeクラスのIsSubclassOfメソッドを使用します。
1 2 3 4 5 6 |
SubClass subClass = new SubClass(); Type type = subClass.GetType(); if (type.IsSubclassOf(typeof(SuperClass))) { Console.WriteLine("SubClass is subclass of BaseClass"); } |
実装しているインターフェースの一覧を取得する
実装しているインターフェースの一覧を取得するには、TypeクラスのGetInterfacesメソッドを使用します。
1 2 3 |
List<int> intList = new List<int>(); Type type = intList.GetType(); Type[] interfaces = type.GetInterfaces(); |
パブリックフィールドを取得する
パブリックフィールドを取得するには、TypeクラスのGetFieldsメソッドを使用します。
1 2 |
Type type = typeof(YourClass); FieldInfo[] fieldInfos = type.GetFields(); |
パブリックプロパティを取得する
パブリックプロパティを取得するには、TypeクラスのGetPropertiesメソッドを使用します。
1 2 |
Type type = typeof(YourClass); PropertyInfo[] propertyInfos = type.GetProperties(); |
パブリックメソッドを取得する
パブリックメソッドを取得するには、TypeクラスのGetMethodsメソッドを使用します。
1 2 |
Type type = typeof(YourClass); MethodInfo[] methodInfos = type.GetMethods(); |
インスタンスフィールドを取得する
インスタンスフィールドを取得するには、TypeクラスのGetFieldsメソッドの引数に、BindingFlags列挙型のInstanceとPublicを指定します。
1 2 |
Type type = typeof(YourClass); FieldInfo[] fieldInfos = type.GetFields(BindingFlags.Instance | BindingFlags.Public); |
インスタンスプロパティを取得する
インスタンスプロパティを取得するには、TypeクラスのGetPropertiesメソッドの引数に、BindingFlags列挙型のInstanceとPublicを指定します。
1 2 |
Type type = typeof(YourClass); PropertyInfo[] propertyInfos = type.GetProperties(BindingFlags.Instance | BindingFlags.Public); |
インスタンスメソッドを取得する
インスタンスメソッドを取得するには、TypeクラスのGetMethodsメソッドの引数に、BindingFlags列挙型のInstanceとPublicを指定します。
1 2 |
Type type = typeof(YourClass); MethodInfo[] methodInfos = type.GetMethods(BindingFlags.Instance | BindingFlags.Public); |
静的フィールドを取得する
静的フィールドを取得するには、TypeクラスのGetFieldsメソッドの引数に、BindingFlags列挙型のStaticとPublicを指定します。
1 2 |
Type type = typeof(YourClass); FieldInfo[] fieldInfos = type.GetFields(BindingFlags.Static | BindingFlags.Public); |
静的プロパティを取得する
静的プロパティを取得するには、TypeクラスのGetPropertiesメソッドの引数に、BindingFlags列挙型のStaticとPublicを指定します。
1 2 |
Type type = typeof(YourClass); PropertyInfo[] propertyInfos = type.GetProperties(BindingFlags.Static | BindingFlags.Public); |
静的メソッドを取得する
静的メソッドを取得するには、TypeクラスのGetMethodsメソッドの引数に、BindingFlags列挙型のStaticとPublicを指定します。
1 2 |
Type type = typeof(YourClass); MethodInfo[] methodInfos = type.GetMethods(BindingFlags.Static | BindingFlags.Public); |
非パブリックフィールドを取得する
非パブリックフィールドを取得するには、TypeクラスのGetFieldsメソッドの引数に、BindingFlags列挙型のNonPublicを指定します。
1 2 3 |
Type type = typeof(YourClass); FieldInfo[] instanceFieldInfos = type.GetFields(BindingFlags.Instance | BindingFlags.NonPublic); FieldInfo[] staticFieldInfos = type.GetFields(BindingFlags.Static | BindingFlags.NonPublic); |
非パブリックプロパティを取得する
非パブリックプロパティを取得するには、TypeクラスのGetPropertiesメソッドの引数に、BindingFlags列挙型のNonPublicを指定します。
1 2 3 |
Type type = typeof(YourClass); PropertyInfo[] instancePropertyInfos = type.GetProperties(BindingFlags.Instance | BindingFlags.NonPublic); PropertyInfo[] staticPropertyInfos = type.GetProperties(BindingFlags.Static | BindingFlags.NonPublic); |
非パブリックメソッドを取得する
非パブリックメソッドを取得するには、TypeクラスのGetMethodsメソッドの引数に、BindingFlags列挙型のNonPublicを指定します。
1 2 3 |
Type type = typeof(YourClass); MethodInfo[] instanceMethodInfos = type.GetMethods(BindingFlags.Instance | BindingFlags.NonPublic); MethodInfo[] staticMethodInfos = type.GetMethods(BindingFlags.Static | BindingFlags.NonPublic); |
現在実行されているメソッドを取得する
現在実行されているメソッドを取得するには、MethodBaseクラスのGetCurrentMethod静的メソッドを使用します。
1 |
MethodBase methodBase = MethodBase.GetCurrentMethod(); |
メソッド名を取得する
メソッド名を取得するには、MethodBaseクラスのNamePropertyを使用します。
1 2 3 4 5 6 |
private void ExampleMethod() { MethodBase methodBase = MethodBase.GetCurrentMethod(); Console.WriteLine(methodBase.Name); // ExampleMethod } |
メソッドが定義されているクラスを取得する
メソッドが定義されているクラス(の型)を取得するには、MethodBaseクラスのDeclaringTypeプロパティを使用します。
1 2 3 4 5 |
MethodBase methodBase = MethodBase.GetCurrentMethod(); Type classType = methodBase.DeclaringType; string className = classType.FullName; Console.WriteLine(className); // ConsoleApp1.Program |
クラスで定義されたメソッドのみ取得する
クラスで定義されたメソッドのみ取得するには、TypeクラスのGetMethodsで取得したメソッド(MethodInfo)のうち、DeclaringTypeプロパティがクラスの型と一致するメソッドのみ抜き出します。
1 2 3 4 5 6 7 8 9 10 11 12 |
Type type = typeof(SubClass); MethodInfo[] methodInfos = type.GetMethods(BindingFlags.Instance | BindingFlags.Public); IEnumerable<MethodInfo> classMethodInfos = methodInfos.Where(x => x.DeclaringType == type); foreach (MethodInfo methodInfo in classMethodInfos) { Console.WriteLine(methodInfo); } /* System.String get_DerivedProperty() Void set_DerivedProperty(System.String) Void DerivedMethod() */ |
特定のフィールドを取得する
特定のフィールドを取得するには、TypeクラスのGetFieldメソッドを使用します。
1 2 3 4 |
Type type = typeof(YourClass); FieldInfo fieldInfo = type.GetField("PublicField"); Console.WriteLine(fieldInfo); // System.String PublicField |
特定のプロパティを取得する
特定のプロパティを取得するには、TypeクラスのGetPropertyメソッドを使用します。
1 2 3 4 |
Type type = typeof(YourClass); PropertyInfo propertyInfo = type.GetProperty("PublicProperty"); Console.WriteLine(propertyInfo); // System.DateTime PublicProperty |
特定のメソッドを取得する
特定のメソッドを取得するには、
1 2 3 4 |
Type type = typeof(YourClass); MethodInfo methodInfo = type.GetMethod("PublicMethod"); Console.WriteLine(methodInfo); // Void PublicMethod() |
インスタンス・メソッドを呼び出す(引数なし)
インスタンス・メソッドを引数なしで呼び出すには、MethodInfoクラスのInvokeメソッドを使用します。
1 2 3 4 5 |
MyClass myClass = new MyClass("名前"); Type type = myClass.GetType(); MethodInfo methodInfo = type.GetMethod("WriteName"); methodInfo.Invoke(myClass, null); // My name is 名前 |
インスタンス・メソッドを呼び出す(引数あり)
インスタンス・メソッドを引数ありで呼び出すには、MethodInfoクラスのInvokeメソッドのパラメーター(引数)に、呼び出すメソッドのパラメーター(引数)を指定します。
1 2 3 4 5 |
MyClass myClass = new MyClass(); Type type = myClass.GetType(); MethodInfo methodInfo = type.GetMethod("WriteNameAndValue"); methodInfo.Invoke(myClass, new object[] { "名前", int.MaxValue }); // Name: 名前, Value: 2147483647 |
静的メソッドを呼び出す(引数なし)
静的メソッドを引数なしで呼び出すには、MethodInfoクラスのInvokeメソッドのパラメーター(引数)に、Nullを指定します。
1 2 3 4 |
Type type = typeof(YourClass); MethodInfo method = type.GetMethod("StaticMethod"); method.Invoke(null, null); // StaticMethod |
静的メソッドを呼び出す(引数あり)
静的メソッドを引数ありで呼び出すには、MethodInfoクラスのInvokeメソッドのパラメーター(引数)に、Nullと呼び出すメソッドのパラメーター(引数)を指定します。
1 2 3 4 |
Type type = typeof(YourClass); MethodInfo method = type.GetMethod("StaticMethodWithParameters"); method.Invoke(null, new object[] { "abcdefghi", 123456789 }); // Param1: abcdefghi, Param2: 123456789 |
メソッドのパラメーター(引数)を取得する
メソッドのパラメーター(引数)を取得するには、MethodInfoクラスのGetParametersメソッドを使用します。
1 2 3 4 5 6 7 8 |
Type type = typeof(ArrayList); MethodInfo methodInfo = type.GetMethod("Add"); ParameterInfo[] parameterInfos = methodInfo.GetParameters(); foreach (ParameterInfo parameterInfo in parameterInfos) { Console.WriteLine(parameterInfo); } // System.Object value |
メソッドの戻り値を取得する
メソッドの戻り値を取得するには、MethodInfoクラスのReturnParameterプロパティを使用します。
1 2 3 4 5 |
Type type = typeof(ArrayList); MethodInfo methodInfo = type.GetMethod("Add"); ParameterInfo parameterInfo = methodInfo.ReturnParameter; Console.WriteLine(parameterInfo); // Int32 |
メソッドの戻り値の型を取得する
メソッドの戻り値の型を取得するには、MethodInfoクラスのReturnTypeプロパティを使用します。
1 2 3 4 5 |
Type type = typeof(MyClass); MethodInfo methodInfo = type.GetMethod("GetTextValue"); Type returnType = methodInfo.ReturnType; Console.WriteLine(returnType); // System.String |
プロパティの型を取得する
プロパティの型を取得するには、PropertyInfoクラスのPropertyTypeプロパティを使用します。
1 2 3 4 5 |
Type type = typeof(MyClass); PropertyInfo propertyInfo = type.GetProperty("Name"); Type propertyType = propertyInfo.PropertyType; Console.WriteLine(propertyType); // System.String |
プロパティの値を取得する
プロパティの値を取得するには、PropertyInfoクラスのGetValueメソッドを使用します。
1 2 3 4 5 6 |
MyClass myClass = new MyClass("MyClassに指定した名前"); Type type = myClass.GetType(); PropertyInfo propertyInfo = type.GetProperty("Name"); object propertyValue = propertyInfo.GetValue(myClass); Console.WriteLine(propertyValue); // MyClassに指定した名前 |
プロパティに値を設定する
プロパティに値を設定するには、PropertyInfoクラスのSetValueメソッドを使用します。
1 2 3 4 5 6 7 |
MyClass myClass = new MyClass(); Type type = myClass.GetType(); PropertyInfo propertyInfo = type.GetProperty("Name"); propertyInfo.SetValue(myClass, "SetValueメソッドで設定する名前"); object propertyValue = propertyInfo.GetValue(myClass); Console.WriteLine(propertyValue); // SetValueメソッドで設定する名前 |
読み取り可能なプロパティかどうかを判定する
読み込み可能なプロパティかどうかを判定するには、PropertyInfoクラスのCanReadプロパティを使用します。
1 2 3 4 5 6 7 |
Type type = typeof(MyClass); PropertyInfo propertyForGet = type.GetProperty("PropertyForGet"); Console.WriteLine(propertyForGet.CanRead); // True PropertyInfo propertyForSet = type.GetProperty("PropertyForSet"); Console.WriteLine(propertyForSet.CanRead); // False |
書き込み可能なプロパティかどうかを判定する
書き込み可能なプロパティかどうかを判定するには、PropertyInfoクラスのCanWriteプロパティを使用します。
1 2 3 4 5 6 7 |
Type type = typeof(MyClass); PropertyInfo propertyForGet = type.GetProperty("PropertyForGet"); Console.WriteLine(propertyForGet.CanWrite); // False PropertyInfo propertyForSet = type.GetProperty("PropertyForSet"); Console.WriteLine(propertyForSet.CanWrite); // True |
プロパティがNull許容型(Nullable<T>型)かどうかを判定する
プロパティがNullable<T>型かどうかを判定するには、TypeクラスのGetGenericTypeDefinitionメソッドの戻り値が、Nullable<>型かどうかを検査します。
1 2 3 4 5 6 7 |
Type type = typeof(MyClass); PropertyInfo prop = type.GetProperty("NullableProperty"); Type propertyType = prop.PropertyType; if (propertyType.GetGenericTypeDefinition() == typeof(Nullable<>)) { Console.WriteLine("aProperty is Nullable<T>."); } |
指定した属性が関連付けられているかどうかを判定する
指定した属性が関連付けられているかどうかを判定するには、TypeクラスのIsDefinedメソッドを使用します。
1 2 3 4 |
Type type = typeof(OurClass); bool isDefined = type.IsDefined(typeof(SerializableAttribute)); Console.WriteLine(isDefined); // True |
型に関連付けられている属性を取得する
型に関連付けられている属性を取得するには、TypeクラスのAttributesプロパティを使用します。
1 2 |
Type type = typeof(OurClass); TypeAttributes attributes = type.Attributes; |
型に関連付けられているカスタム属性を含むコレクションを取得する
型に関連付けられているカスタム属性を含むコレクションを取得するには、TypeクラスのCustomAttributesプロパティ、またはGetCustomAttributesDataメソッドを使用します。
1 2 3 |
Type type = typeof(OurClass); IEnumerable<CustomAttributeData> customAttributes = type.CustomAttributes; IList<CustomAttributeData> customAttributeDataList = type.GetCustomAttributesData(); |
型に関連付けられている特定の型の属性を取得する
クラスに付加された特定の属性を取得するには、TypeクラスのGetCustomAttributeメソッド、またはGetCustomAttribute<T>メソッドを使用します。
1 2 3 |
Type type = typeof(OurClass); Attribute customAttribute = type.GetCustomAttribute(typeof(SerializableAttribute)); SerializableAttribute customAttributeGeneric = type.GetCustomAttribute<SerializableAttribute>(); |
プロパティに関連付けられている属性を取得する
型に関連付けられている属性を取得するには、PropertyInfoクラスのAttributesプロパティを使用します。
1 2 3 |
Type type = typeof(OurClass); PropertyInfo propertyInfo = type.GetProperty("OurProperty"); PropertyAttributes attributes = propertyInfo.Attributes; |
プロパティに関連付けられているカスタム属性を含むコレクションを取得する
プロパティに関連付けられているカスタム属性を含むコレクションを取得するには、PropertyInfoクラスのCustomAttributesプロパティ、またはGetCustomAttributesDataメソッドを使用します。
1 2 3 4 |
Type type = typeof(OurClass); PropertyInfo propertyInfo = type.GetProperty("OurProperty"); IEnumerable<CustomAttributeData> customAttributes = propertyInfo.CustomAttributes; IList<CustomAttributeData> customAttributeDataList = propertyInfo.GetCustomAttributesData(); |
プロパティに関連付けられている特定の型の属性を取得する
プロパティに関連付けられている特定の型の属性を取得するには、PropertyInfoクラスのGetCustomAttributeメソッド、またはGetCustomAttribute<T>メソッドを使用します。
1 2 3 4 |
Type type = typeof(OurClass); PropertyInfo propertyInfo = type.GetProperty("OurProperty"); Attribute customAttribute = propertyInfo.GetCustomAttribute(typeof(IsCustomAttribute)); IsCustomAttribute customAttributeGeneric = propertyInfo.GetCustomAttribute<IsCustomAttribute>(); |
メソッドに関連付けられている属性を取得する
型に関連付けられている属性を取得するには、MethodInfoクラスのAttributesプロパティを使用します。
1 2 3 |
Type type = typeof(OurClass); MethodInfo methodInfo = type.GetMethod("OurMethod"); MethodAttributes attributes = methodInfo.Attributes; |
メソッドに関連付けられているカスタム属性を含むコレクションを取得する
メソッドに関連付けられているカスタム属性を含むコレクションを取得するには、MethodInfoクラスのCustomAttributesプロパティ、またはGetCustomAttributesDataメソッドを使用します。
1 2 3 4 |
Type type = typeof(OurClass); MethodInfo methodInfo = type.GetMethod("OurMethod"); IEnumerable<CustomAttributeData> customAttributes = methodInfo.CustomAttributes; IList<CustomAttributeData> customAttributeDataList = methodInfo.GetCustomAttributesData(); |
メソッドに関連付けられている特定の型の属性を取得する
メソッドに関連付けられている特定の型の属性を取得するには、MethodInfoクラスのGetCustomAttributeメソッド、またはGetCustomAttribute<T>メソッドを使用します。
1 2 3 4 |
Type type = typeof(OurClass); MethodInfo methodInfo = type.GetMethod("OurMethod"); Attribute customAttribute = methodInfo.GetCustomAttribute(typeof(ObsoleteAttribute)); ObsoleteAttribute customAttributeGeneric = methodInfo.GetCustomAttribute<ObsoleteAttribute>(); |
型のメンバーを取得する
型のメンバーを取得するには、TypeクラスのGetMembersメソッドを使用します。
1 2 |
Type type = typeof(Form); MemberInfo[] memberInfos = type.GetMembers(); |
名前を指定して型のメンバーを取得する
名前を指定して型のメンバーを取得するには、TypeクラスのGetMemberメソッドを使用します。
1 2 |
Type type = typeof(Form); MemberInfo[] memberInfos = type.GetMember("ShowDialog"); |
メンバーの種類を取得する
メンバーの種類を取得するには、TypeクラスのGetMemberメソッドを使用します。
1 2 3 4 5 6 7 8 |
Type type = typeof(Form); MemberInfo[] memberInfos = type.GetMember("Click"); foreach (MemberInfo memberInfo in memberInfos) { MemberTypes memberTypes = memberInfo.MemberType; Console.WriteLine(memberTypes); } // Event |
すべてのフィールドを取得する
すべてのフィールドを取得するには、TypeクラスのGetRuntimeFieldsメソッドを使用します。
1 2 |
Type type = typeof(Form); IEnumerable<FieldInfo> fieldInfos = type.GetRuntimeFields(); |
すべてのプロパティを取得する
すべてのプロパティを取得するには、TypeクラスのGetRuntimePropertiesメソッドを使用します。
1 2 |
Type type = typeof(Form); IEnumerable<PropertyInfo> propertyInfos = type.GetRuntimeProperties(); |
すべてのメソッドを取得する
すべてのメソッドを取得するには、TypeクラスのGetRuntimeMethodsメソッドを使用します。
1 2 |
Type type = typeof(Form); IEnumerable<MethodInfo> methodInfos = type.GetRuntimeMethods(); |
すべてのイベントを取得する
すべてのイベントを取得するには、TypeクラスのGetRuntimeEventsメソッドを使用します。
1 2 |
Type type = typeof(Form); IEnumerable<EventInfo> eventInfos = type.GetRuntimeEvents(); |