.NETにはリフレクション(System.Reflection名前空間)という、アセンブリや、アセンブリに定義されているクラス、インターフェース、構造体、列挙体などに関する情報を取得できる仕組みが用意されています。
リフレクションを使うとプログラムの実行時に型のインスタンスを作成したり、作成した型インスタンスを呼び出したり、アクセスしたりすることができます。
今回は、リフレクション(Reflection)を使用して、クラスや構造体などのオブジェクトからプロパティの一覧を取得する方法を紹介します。
オブジェクトの型を取得する
オブジェクトからプロパティの一覧を取得するには、まず型(Type)を取得します。
1 2 3 4 5 6 7 8 9 |
// 以下のusingが必要 // using System; // using System.Text; // StringBuilderクラスの型を取得 Type typeOfStringBuilder = typeof(StringBuilder); // DateTime構造体の型を取得 Type typeOfDateTime = typeof(DateTime); |
オブジェクトの型オブジェクトを取得する方法については、以下の記事を参考にしてください。
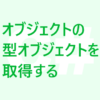
プロパティの一覧を取得する
プロパティの一覧を取得するには、TypeクラスのGetPropertiesメソッドを使用します。
1 2 3 4 5 6 7 8 9 |
// 以下のusingが必要 // using System; // using System.Reflection; // using System.Collections.Generic; List<string> stringList = new List<string>(); Type typeOfListString = stringList.GetType(); // List<string>オブジェクトのプロパティの一覧を取得 PropertyInfo[] propertyInfos = typeOfListString.GetProperties(); |
GetPropertiesメソッドはパラメーター(引数)を指定せずに呼び出すと、すべてのパブリックプロパティを返します。
GetPropertiesメソッドには、取得するプロパティの種類を表すフラグ(メンバーと型の検索方法を制御するフラグ)を指定することができます。
指定するフラグには、System.Reflection名前空間にあるBindingFlags列挙型のフィールド値を指定します。
BindingFlags列挙型には、パブリックメンバーを表す「Public」、パブリック以外のメンバーを表す「NonPublic」や、インスタンスメンバーを表す「Instance」、静的メンバーを表す「Static」などがあります。
以下にColor構造体から静的パブリックプロパティの一覧と、インスタンス非パブリックプロパティの一覧を取得する例を示します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
// 以下のusingが必要 // using System; // using System.Drawing; // using System.Reflection; // 型を取得 Type type = typeof(Color); // 静的パブリックプロパティの一覧を取得 PropertyInfo[] staticPublicProperties = type.GetProperties(BindingFlags.Static | BindingFlags.Public); // 一覧を列挙 foreach (PropertyInfo propertyInfo in staticPublicProperties) { // プロパティ名を取得 string name = propertyInfo.Name; Console.WriteLine(name); } /* Transparent AliceBlue AntiqueWhite Aqua ... 中略 ... WhiteSmoke Yellow YellowGreen */ // インスタンスパブリック以外プロパティの一覧を取得 PropertyInfo[] instanceNonPublicProperties = type.GetProperties(BindingFlags.Instance | BindingFlags.NonPublic); // 一覧を列挙 foreach (PropertyInfo propertyInfo in instanceNonPublicProperties) { // プロパティ名を取得 string name = propertyInfo.Name; Console.WriteLine(name); } /* NameAndARGBValue Value */ |
特定のプロパティを取得する
GetPropertiesメソッドではプロパティの一覧が取得できますが、Typeクラスには特定のプロパティの情報を取得するメソッドも用意されています。
特定のプロパティの情報を取得するにはGetPropertyメソッドを使用します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// 以下のusingが必要 // using System; // using System.Data; DataSet dataSet = new DataSet("SampleDataSet"); Type type = dataSet.GetType(); // DataSetクラスのTablesプロパティの情報を取得 PropertyInfo propertyInfo = type.GetProperty("Tables"); // プロパティタイプを出力 Type propertyType = propertyInfo.PropertyType; Console.WriteLine(propertyType.FullName); // System.Data.DataTableCollection |
GetPropertyメソッドで特定のプロパティの情報を取得するには、メソッドのパラメーター(引数)にプロパティ名を指定します。
GetPropertyメソッドのパラメーター(引数)にプロパティ名のみ指定した場合は、パブリックプロパティを対象に情報を取得します。
パブリックプロパティ以外のプロパティや、インスタンスプロパティ、静的プロパティなどのプロパティの形式を指定してGetPropertyメソッドを呼び出す場合は、GetPropertiesメソッドと同様にパラメーターにBindingFlags列挙型の値を指定します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
// 以下のusingが必要 // using System; // using System.Data; DataSet dataSet = new DataSet("SampleDataSet"); Type type = dataSet.GetType(); // DataSetクラスのTablesプロパティの情報を取得 PropertyInfo propertyInfo = type.GetProperty( "Tables", BindingFlags.Instance | BindingFlags.Public); // プロパティタイプを出力 Type propertyType = propertyInfo.PropertyType; Console.WriteLine(propertyType.FullName); // System.Data.DataTableCollection |
サンプルプログラム
サンプルとしてWindowsフォームアプリケーションで、指定された文字列からオブジェクトの型(Type)を取得してプロパティの一覧を取得し、プロパティ名を表示するプログラムを作成します。
ユーザーインターフェース
Visual StudioでWindowsフォームアプリケーションのプロジェクトを作成し、フォームに型名を入力するテキストボックス(textBox1)と、プロパティの一覧を取得して、名前を表示するボタン(button1)と、プロパティ名の一覧を表示するテキストボックス(textBox2)を配置します。
ソースコード
ボタンクリック時のイベント処理(オブジェクトのプロパティの一覧を表示する処理)を記述します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
using System; using System.Drawing; using System.Text; using System.Windows.Forms; // System.Reflectionのusingを追加 using System.Reflection; namespace WindowsFormsApp1 { public partial class Form1 : Form { // コンストラクタ public Form1() { InitializeComponent(); } // プロパティの一覧を取得する private void button1_Click(object sender, EventArgs e) { try { // タイプを表す文字列(名前)を取得 string typeName = this.textBox1.Text; // タイプに変換 Type type = Type.GetType(typeName); // プロパティの一覧を取得 PropertyInfo[] propertyInfos = type.GetProperties( BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.Instance | BindingFlags.Static ); StringBuilder stringBuilder = new StringBuilder(); // プロパティを列挙 foreach (PropertyInfo propertyInfo in propertyInfos) { stringBuilder.AppendLine(propertyInfo.Name); } // プロパティの一覧を表示 this.textBox2.Text = stringBuilder.ToString(); } catch (Exception ex) { MessageBox.Show(ex.Message); } } } } |
プログラムの実行
プロジェクトをビルドして実行(デバッグ)します。
オブジェクトのタイプ名をテキストボックスに入力します。
ここではタイプ名に「System.String」を入力しています。
ボタンをクリックします。
プロパティの一覧が取得されてテキストボックスに表示されます。
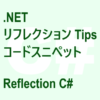